Customizing Linux filesystem commands

The power of the Linux terminal is that you can quickly customize your environment so that it works better and harder according to your needs. There are many different ways to "hack" your terminal, some of which are practically entire fields of computer science in themselves, but there are lots of small tricks for beginners—especially when it comes to working with the Linux filesystem. See my article Managing files with the Linux terminal for the basics.
The simplest way to customize your working terminal environment is by editing its configuration file, which contains your personal shell settings. Bash (the Bourne Again Shell) is the most common shell on Linux (Red Hat Enterprise Linux and Fedora included), and Bash's configuration file is ~/.bashrc
.
Create an alias
An easy first step into customization is creating aliases. Aliases allow you to define your own simple commands or redefine existing ones. To add aliases, edit your file .bashrc
in a text editor, or create them directly from the terminal using the echo
command.
For example, to redefine the ls
command so that it always uses the --classify
(-F
) and --almost-all
(-a
) options, add an alias rule to .bashrc
, and then source
(re-read and load) the altered configuration file.
$ echo "alias ls='ls --classify --almost-all'" >> ~/.bashrc
$ source .bashrc
$ ls
bin/
Desktop/
despacer.sh
documentation.zip*
Documents/
Music/
Pictures/
Public/
Once the alias has been created and re-loaded, the ls
command will always use the --classify
option so you can distinguish files from folders, and the --almost-all
option to exclude the dot and double-dot notation. There are many more options for the ls
command. See the ls
manual for more information by typing:
$ man ls
You can create aliases for all manner of repetitious simple tasks. Complex operations may require something more robust than an alias, like a shell script, but aliases are a great way to let your environment work for you.
Customize your trash
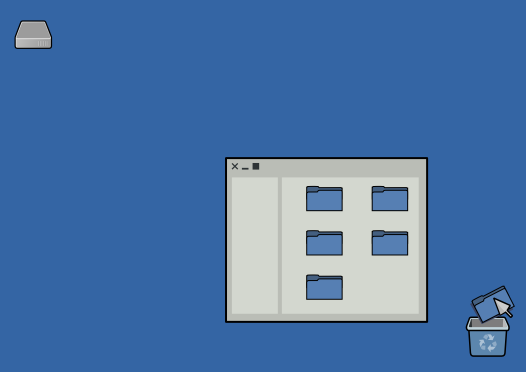
Open a series of windows to find the file you want to trash. Move the file to the Trash or Bin icon on your Desktop. When you've collected enough trash and are confident that it all can be safely removed from your system, go to the Trash menu and select Empty.
There is no trash bin in your terminal by default, but then again, a "trash bin" is just another folder that accepts all of the files you intend to eventually delete. The data is still there until you empty it. Doing so is trivial. With the lessons you've learned in this article, you can create a safe and reliable trash system for your terminal.
If your computer happens to have a desktop, such as the GNOME desktop on Red Hat Enterprise Linux and Fedora, then you already have a system trash bin located at ~/.local/share/Trash/files
. You can reference this folder directly, or to reduce typing you can create a symlink to it:
$ ln --symbolic ~/.local/share/Trash/files ~/.trash
If your computer has no desktop, then you may not have a Trash folder, but you can create one manually. A trash bin is really just another folder. Use the mkdir
command with the --parents
(-p
) option to create a series of folders all in one command:
$ mkdir --parents ~/.local/share/Trash/files
$ ln --symbolic ~/.local/share/Trash/files ~/.trash
When you want to move a file or a folder to the trash bin, use the mv
command as usual:
$ mv oldfile.txt ~/.trash
Make a better trash command
An even smoother way of using a Trash folder is to write your own new command called trash
, which moves a file to your ~/.trash
folder. This is an advanced exercise and introduces a few new commands, but you might be surprised at how easy it is to create your own custom commands. In just two lines of Bash code, you can design your own personal system of waste management.
Before you create your own command, you need a place to store custom scripts. It's common for Linux users to maintain a ~/bin
directory, where they keep personal utilities and binary executables. This doesn't exist by default, but you can create it and then add it to your path:
$ mkdir ~/bin
$ echo "export PATH=$PATH:$HOME/bin" >> ~/.bashrc
$ source ~/.bashrc
Now you can build your own trash command with the echo
command:
$ echo '#!/bin/sh' > ~/trash
$ echo 'mv "${@}" ~/.local/share/Trash/files' >> ~/trash
Alternately, you can open a text editor and copy and paste the code into it:
#!/bin/sh
mv "${@}" ~/.local/share/Trash/files
Save the file as ~/bin/trash.
This script's first line declares what application is required to run it. In this case, the first line specifies that the sh
shell, located in the /bin
directory at the root of your filesytem, is required. After all, this is a shell script: a series of Bash shell commands in the order of desired execution.
The second line uses the mv
command in conjunction with a special Bash variable, "${@}"
, meaning anything typed after the command. This is what makes it possible for a single command to act on several files. You won't have to run your command once per file like this:
$ trash foo
$ trash bar
$ trash baz
Instead, you're programming your command to recognize several arguments at once:
$ trash foo bar baz
To confirm that your shell script is correct, use the cat
command to concatenate the file's contents into your shell's output:
$ cat trash
#!/bin/sh
mv "${@}" ~/.local/share/Trash/files
To make this command usable for yourself, use mv
to move it into a directory called ~/bin
(this directory doesn't exist by default, so create it first):
$ mkdir ~/bin
$ mv trash ~/bin
Your shell currently sees your shell script as a file full of text. You haven't yet given permission for the computer to treat your script as an executable command. This differentiation is important, because if your shell was able to execute everything as a command without your explicit permission, then any file on your system would have the potential of executing arbitrary code (imagine dropping all tables in an important database just because you or one of your users clicked on the wrong file).
To grant a file permission to be treated as an executable, you set the executable bit in its file attributes using the chmod
(change mode) command:
$ chmod +x ~/bin/trash
Now you can run the file as a command. Test this by creating a file and then moving that file to the trash:
$ echo "This is rubbish." >> rubbish.file
$ cat rubbish.file
This is rubbish.
$ ~/bin/trash rubbish.file
If you're running a desktop on your computer, open up your trash bin and confirm that rubbish.file
is now in your system trash. If you're not running a desktop, just look into your ~/.trash
symlink. As an ad hoc filter, send the output of the ls
command through a pipe (|
), using the grep
command to search for the string rubbish:
$ ls ~/.trash | grep rubbish
rubbish.file
Notice, however, that using your trash
command without providing the full path does not work as expected:
$ echo "This is more rubbish." >> rubbish2.file
$ trash rubbish2.file
bash: trash: command not found...
This is because it is not yet in your executable path.
Tell your shell where to look
Some commands, like cd
, pwd
, and alias
, are built into your shell. Others, like ls
, mv
, and echo
, are installed in locations your shell designates as valid search locations for commands. When you type in commands like ls
, mv
, or trash
, your shell searches through a list of directories that it has been told contain executable files. You can view this list of search locations by looking at the PATH
variable. The echo
command lets you look into variables by prepending a dollar sign ($
) before the variable's name:
$ echo $PATH
/usr/bin:/usr/sbin:/bin:/sbin:/usr/local/bin:/usr/local/sbin
So that you can use trash
just as you would any other command on your system, you must add your personal ~/bin
directory to your PATH variable. Shell environment variables are loaded when the shell is launched, so they're set in the ~/.bashrc
configuration file. You can add this directory into your PATH using a text editor, or just use the echo
command with a double redirect to append the rule to your configuration:
$ echo 'export PATH=$PATH:/home/seth/bin' >> ~/.bashrc
Load your new configuration into your shell:
$ source ~/.bashrc
And try your command one more time:
$ trash rubbish2.file
Permanently remove a file
Eventually, your trash bin needs emptying. The rm
command removes a file from your filesystem (permanently, so use it sparingly and carefully).
Because the rm
command can be harmful if misused, it's a good idea to limit your interaction with it, and to limit its scope when using it. In this case, you want rm
to only operate within your trash bin, so you can create an alias to reduce the risk of typing the command wrong. The fewer times you invoke rm
, the less chance you have to accidentally remove files.
Create an alias called empty
by typing the following:
$ echo 'empty="rm --recursive --verbose --force ~/.local/share/Trash/files/*"' >> ~/.bashrc
Unlike your trash
command, empty
is treated as a built-in command since it's an alias rather than an external script. That means you don't have to put anything into your ~/bin
directory or add anything to your PATH
variable. You only need to reload your shell configuration, and then test out your new built-in command:
$ source .bashrc
$ empty
removed '/home/seth/.trash/rubbish.file
removed '/home/seth/.trash/rubbish2.file
Practice makes fluent
You have now programmed your first shell script, and customized your shell environment. Small exercises such as this one are important, because the more you work in the shell, the better at it you become. Try to maximize your time working in the terminal to get comfortable with it. You don't have to do major tasks at first. Start small, and work your way up.
There's no better time like the present, so go explore the Linux terminal!
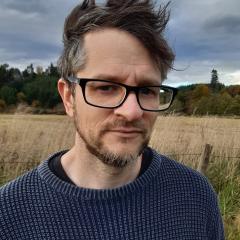
Seth Kenlon
Seth Kenlon is a UNIX geek and free software enthusiast. More about me